今天晚上下载了几个英语听力拷贝到了MP3上听,可是只听看不到原文真是蛋疼,所以想把听力原文做成lrc文件,这样MP3就能显示歌词了。本来想上网上下载个,突然一想这程序也不难,就动手自己写了个,晒下..有BUG,今后会不断改进并添加新功能~

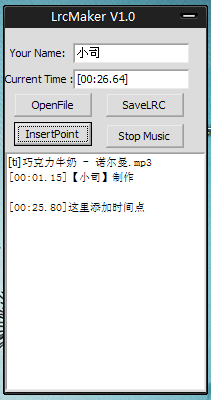


使用方法:
先在your name里输入你的名字,程序会自动在生成的lrc里添加你的信息~
去网上把歌词copy到下面的文本框内,
点openfile找个mp3,然后Current time里显示的就是当前时间,精确到毫秒。
等听到歌唱到那句话的时间,把光标放到那句的前边,点InsertPoint就行啦~
最后别忘了点SaveLRC!
主要代码:
main.cpp
#ifndef INCLUDE_H_H_H_H
#define INCLUDE_H_H_H_H
#include <windows.h>
#include <stdio.h>
#endif
#include "resource.h"
#include "music.h"
#pragma comment(lib,"Winmm.lib")
long MilliSecond_Count=0;
int MilliSecond=0;
int Second=0;
int Minute=0;
bool IsPlaying=false;
TCHAR szTemp[MAX_PATH];
INT_PTR ExitCode;
int WINAPI WinMain(HINSTANCE hIns,HINSTANCE hPre,LPSTR lpCmd,int nShow)
{
LoadLibrary("Riched20.dll");
ZeroMemory(szTemp,MAX_PATH);
ExitCode=DialogBox(hIns,MAKEINTRESOURCE(IDD_DIALOG1),NULL,DialogProc);
return 0;
}
//窗口过程函数
INT_PTR CALLBACK DialogProc(HWND hwndDlg,UINT uMsg,WPARAM wParam,LPARAM lParam)
{
switch(uMsg)
{
case WM_INITDIALOG:
InitDialog(hwndDlg);
break;
case WM_COMMAND:
if(IDC_OPEN==wParam)
{
OpenFile(hwndDlg);
}
if(IDC_INSERT==wParam)
{
char ch[11];
memset(ch,0,11);
Convent(ch);
HWND hText=GetDlgItem(hwndDlg,IDC_TEXT);
SendMessage(hText,EM_REPLACESEL,FALSE,(WPARAM)ch);
}
if(IDC_STOP==wParam)
{
StopMusic(szTemp,hwndDlg);
if(IDYES==MessageBox(hwndDlg,"Do you want to clean the text ?","Warming",MB_YESNO | MB_ICONQUESTION))
{
SetDlgItemText(hwndDlg,IDC_TEXT,"");
}
}
if(IDC_SAVE==wParam)
{
if(!SaveLRC(hwndDlg))
{
MessageBox(hwndDlg,"Save LRC failed !","Warming",0);
}
}
break;
case WM_CLOSE:
EndDialog(hwndDlg,ExitCode);
break;
default:
break;
}
return FALSE;
}
music.cpp
#include "music.h"
#include "resource.h"
extern long MilliSecond_Count;
extern int MilliSecond;
extern int Second;
extern int Minute;
extern bool IsPlaying;
extern TCHAR szTemp[MAX_PATH];
extern INT_PTR ExitCode;
//显示打开文件对话框
void OpenFile(HWND hwndDlg)
{
OPENFILENAME fn;
ZeroMemory(&fn,sizeof(fn));
TCHAR szPath[MAX_PATH];
ZeroMemory(szPath,MAX_PATH);
TCHAR szTitle[MAX_PATH];
ZeroMemory(szTitle,MAX_PATH);
fn.lStructSize=sizeof(fn);
fn.hwndOwner=hwndDlg;
fn.lpstrFilter="MP3音乐文件(*.mp3)\0*.mp3\0";
fn.lpstrFile=szPath;
fn.nMaxFile=MAX_PATH;
fn.lpstrFileTitle=szTitle;
fn.nMaxFileTitle=MAX_PATH;
fn.lpstrInitialDir=NULL;
fn.lpstrTitle="Choose a MP3 file :";
fn.Flags=OFN_EXPLORER | OFN_PATHMUSTEXIST | OFN_FILEMUSTEXIST | OFN_OVERWRITEPROMPT;
if(GetOpenFileName(&fn))
{
TCHAR szShortPath[MAX_PATH];
TCHAR szCommand[MAX_PATH+30];
ZeroMemory(szShortPath,MAX_PATH);
ZeroMemory(szCommand,MAX_PATH+30);
GetShortPathName(szPath,szShortPath,MAX_PATH);
//如果正在播放,那么就先停止播放,并清空EDIT中的内容
if(IsPlaying)
{
StopMusic(szShortPath,hwndDlg);
SetDlgItemText(hwndDlg,IDC_TEXT,"");
}
sprintf(szCommand,"play %s",szShortPath);
mciSendString(szCommand,NULL,0,NULL);
SetTimer(hwndDlg,1,1,TimerProc);
IsPlaying=true;
strcpy(szTemp,szShortPath);
AddInfo(hwndDlg,szTitle);
}
}
//Timer的回调函数
void CALLBACK TimerProc(HWND hwnd,UINT uMsg,UINT_PTR idEvent,DWORD dwTime)
{
++MilliSecond;
++MilliSecond_Count;
if(MilliSecond_Count>=GetMusicLength(szTemp)/10)
{
StopMusic(szTemp,hwnd);
}
if(100==MilliSecond)
{
MilliSecond=0;
++Second;
}
if(60==Second)
{
Second=0;
++Minute;
}
char ch[11];
memset(ch,0,11);
Convent(ch);
SetDlgItemText(hwnd,IDC_TIME,ch);
}
//把当前时间转换成 [xx:xx.xx]格式
void Convent(char *pResult)
{
char bufMinute[3];
char bufSecond[3];
char bufMilli[3];
if(Minute<10)
{
bufMinute[0]='0';
itoa(Minute,&bufMinute[1],10);
}
else if(Minute>=10)
{
itoa(Minute,bufMinute,10);
}
if(Second<10)
{
bufSecond[0]='0';
itoa(Second,&bufSecond[1],10);
}
else if(Second>=10)
{
itoa(Second,bufSecond,10);
}
if(MilliSecond<10)
{
bufMilli[0]='0';
itoa(MilliSecond,&bufMilli[1],10);
}
else if(MilliSecond>=10)
{
itoa(MilliSecond,bufMilli,10);
}
sprintf(pResult,"[%s:%s.%s]",bufMinute,bufSecond,bufMilli);
}
void InitDialog(HWND hwndDlg)
{
// HWND hText=GetDlgItem(hwndDlg,IDC_TEXT);
// SendMessage(hText,WM_USER + 72,(WPARAM)GetDC(hText),64);
}
//自动添加作者的名字,歌曲的标题
void AddInfo(HWND hwndDlg,char *pName)
{
int Length_Title=GetLength(pName);
int Length_Name=GetWindowTextLength(GetDlgItem(hwndDlg,IDC_NAME));
int Length_Text=GetWindowTextLength(GetDlgItem(hwndDlg,IDC_TEXT));
int Length_All=Length_Title + Length_Name + Length_Text;
char *pBuf=new char[Length_All + 1 + 20];
pBuf[Length_All + 20]='\0';
char *pTitle=new char[Length_Title + 1];
char *pMyName=new char[Length_Name + 1];
char *pText=new char[Length_Text + 1];
pTitle[Length_Title]='\0';
pMyName[Length_Name]='\0';
pText[Length_Text]='\0';
GetDlgItemText(hwndDlg,IDC_NAME,pMyName,Length_Name + 1);
GetDlgItemText(hwndDlg,IDC_TEXT,pText,Length_Text + 1);
strcpy(pTitle,pName);
sprintf(pBuf,"[ti]%s\r\n[00:01.15]【%s】制作\r\n%s",pTitle,pMyName,pText);
SetDlgItemText(hwndDlg,IDC_TEXT,pBuf);
}
int GetLength(char *pCh)
{
int ix=0;
while(pCh[ix]!='\0')
{
++ix;
}
return ix;
}
//显示保存对话框
bool SaveLRC(HWND hwndDlg)
{
OPENFILENAME fn;
char szPath[MAX_PATH];
char szTitle[MAX_PATH];
ZeroMemory(szTitle,MAX_PATH);
ZeroMemory(szPath,MAX_PATH);
ZeroMemory(&fn,sizeof(fn));
fn.lStructSize=sizeof(fn);
fn.hwndOwner=hwndDlg;
fn.lpstrFilter="LRC歌词文件(*.lrc)\0*.lrc\0";
fn.lpstrFile=szPath;
fn.nMaxFile=MAX_PATH;
fn.lpstrFileTitle=szTitle;
fn.nMaxFileTitle=MAX_PATH;
fn.lpstrInitialDir=NULL;
fn.lpstrTitle="Save LRC :";
fn.Flags=OFN_EXPLORER | OFN_PATHMUSTEXIST | OFN_FILEMUSTEXIST | OFN_OVERWRITEPROMPT;
if(GetSaveFileName(&fn))
{
int len=GetWindowTextLength(GetDlgItem(hwndDlg,IDC_TEXT));
char *pBuf=new char[len + 1];
GetDlgItemText(hwndDlg,IDC_TEXT,pBuf,len + 1);
char szFileName[MAX_PATH + 30];
sprintf(szFileName,"%s.lrc",szPath);
FILE *f=NULL;
f=fopen(szFileName,"w");
if(NULL==f)
{
return false;
}
fwrite(pBuf,1,len,f);
fclose(f);
}
return true;
}
//得到音乐的长度
long GetMusicLength(char *pPath)
{
long len=0;
char szLen[256];
memset(szLen,0,256);
char Com[256];
sprintf(Com,"status %s length",szTemp);
mciSendString(Com,szLen,256,NULL);
return strtol(szLen,NULL,10);
}
//停止播放音乐
void StopMusic(char *pPath,HWND hwndDlg)
{
TCHAR szCmd[50];
ZeroMemory(szCmd,50);
sprintf(szCmd,"close %s",szTemp);
mciSendString(szCmd,NULL,0,NULL);
KillTimer(hwndDlg,1);
IsPlaying=false;
InitVar();
}
//变量初始化
void InitVar()
{
MilliSecond_Count=0;
MilliSecond=0;
Second=0;
Minute=0;
}
分享到:
相关推荐
全志平台R11 SDK用户手册,全志R11_Tina_SDK_User_Manual_V1.0,全志R11 Tina linux 用户手册,介绍如何在Tina SDK 的平台上开发应用SDK 的使用 Tins SDK 的编译、打包、内置命令请参考文档《SDK_Quick_start_Guide》 ...
成都市医保局加解密方法工具类及调用demo(医保电子处方中心)V1.0
Hi3521V100SDKV1.0.4.0 Hi3520V400SDKV1.0.4.0
02.HiLink SDK 集成开发checklist_v1.0,hilink智能家居系统
精锐IV单机锁的开发包安装程序,无密码 下载安装后,开发包中包括精锐IV开发手册、各种工具以及开发过程中需要使用的其他资料等。
Hi3518 Software Developer Kit V1.0.4.0.part01.rar
Hi3518 Software Developer Kit V1.0.4.0.part04
Hi3518 Software Developer Kit V1.0.4.0.part08
Hi3518 Software Develorer Kit V1.0.4.0.part02
taobao-sdk-java-auto-1.0.jar,
Hi3559V200_MobileCam_SDK_V1.0.1.5——ReleaseDoc_zh.zip
涂鸦智能WIFI-SDK_2M说明v1.0.01
NuTiny-SDK-N76E003 V1.0.DSN NUTINY-SDK-N76E003 V1.0.DSNlck nutiny-sdk-n76e003 v1.0.opj NuTiny-SDK-N76E003 V1.0.pdf NuTiny-SDK-N76E003 V1.0.eco NuTiny-SDK-N76E003 V1.0.pcb NuTiny-SDK-N76E003 V1.0_BOM....
iPhone SDK编程入门经典,让您轻松入门学习IOS的开发
NEMeeting SDK网易会议 v1.0.zip
本文档主要面向JAVA开发人员,旨在指导JAVA开发人员利用AWS S3 JAVA SDK进行开发,对接XSKY EOS产品。阅 读该文档最好对对象存储有一定的了解,并且详细阅读过《XSKY EOS应用与开发指南》。 文档主要包括以下内容: ...
选择文件 ( Mail_sdk_v1.0.rar ) 灌
The Microsoft Kinect for Windows SDK provides the native and managed APIs and the tools you need to develop Kinect enabled applications for Windows. Applications that are built using the Kinect SDK ...
SDK编程讲座SDK编程讲座SDK编程讲座SDK编程讲座